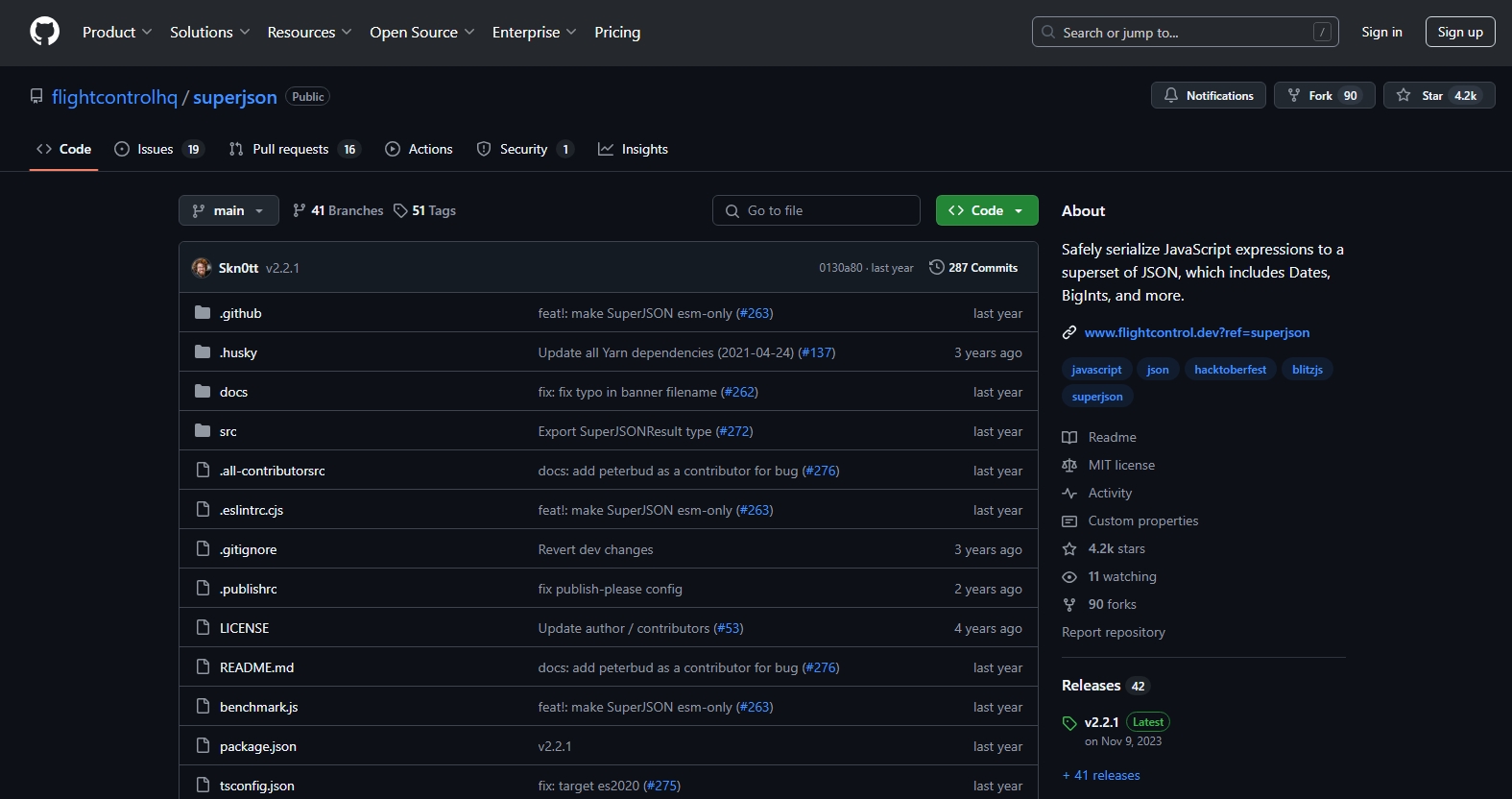

Superjson
Serialize and Deserialize Complex JavaScript Data Types
About this tool
Superjson is a powerful tool designed to handle the serialization and deserialization of complex JavaScript data types. It ensures that when you serialize data, it retains its original type, making it indispensable for applications where data integrity is crucial.
When working with JavaScript, especially in state management and server-side rendering, serialization becomes a critical issue. The built-in `JSON.stringify()` method has limitations, particularly when dealing with complex data types such as `Map`, `Set`, `Date`, and others. This is where Superjson comes into play, providing a robust solution for serializing and deserializing data while preserving its type.
One of the key features of Superjson is its ability to handle complex data structures, making it ideal for modern JavaScript applications. Here’s an example of how Superjson can be used to serialize and deserialize data:
import superjson from 'superjson'; interface BearState { bear: Map; fish: Set; time: Date; query: RegExp; } const storage: PersistStorage = { getItem: (name) => { const str = localStorage.getItem(name); if (!str) return null; return superjson.parse(str); }, setItem: (name, value) => { localStorage.setItem(name, superjson.stringify(value)); }, removeItem: (name) => localStorage.removeItem(name), }; const initialState: BearState = { bear: new Map(), fish: new Set(), time: new Date(), query: new RegExp(''), }; export const useBearStore = create()( persist( (set) => ({ ...initialState, // ... }), ), );
Superjson is particularly useful in frameworks like Next.js or Remix, where the serialization of the `dehydratedState` is handled automatically. However, if you need to return complex types, Superjson is a great choice. Here’s an example:
return { props: { dehydratedState: dehydrate(queryClient) } };
While Superjson is powerful, it’s important to note that it does not escape values, which can lead to XSS vulnerabilities if not handled properly. For custom SSR setups, consider using libraries like Serialize JavaScript or devalue to ensure safety against XSS injections.
Superjson provides a comprehensive solution for serializing complex JavaScript data types, making it an essential tool for modern web development.
🔥 Featured Tools
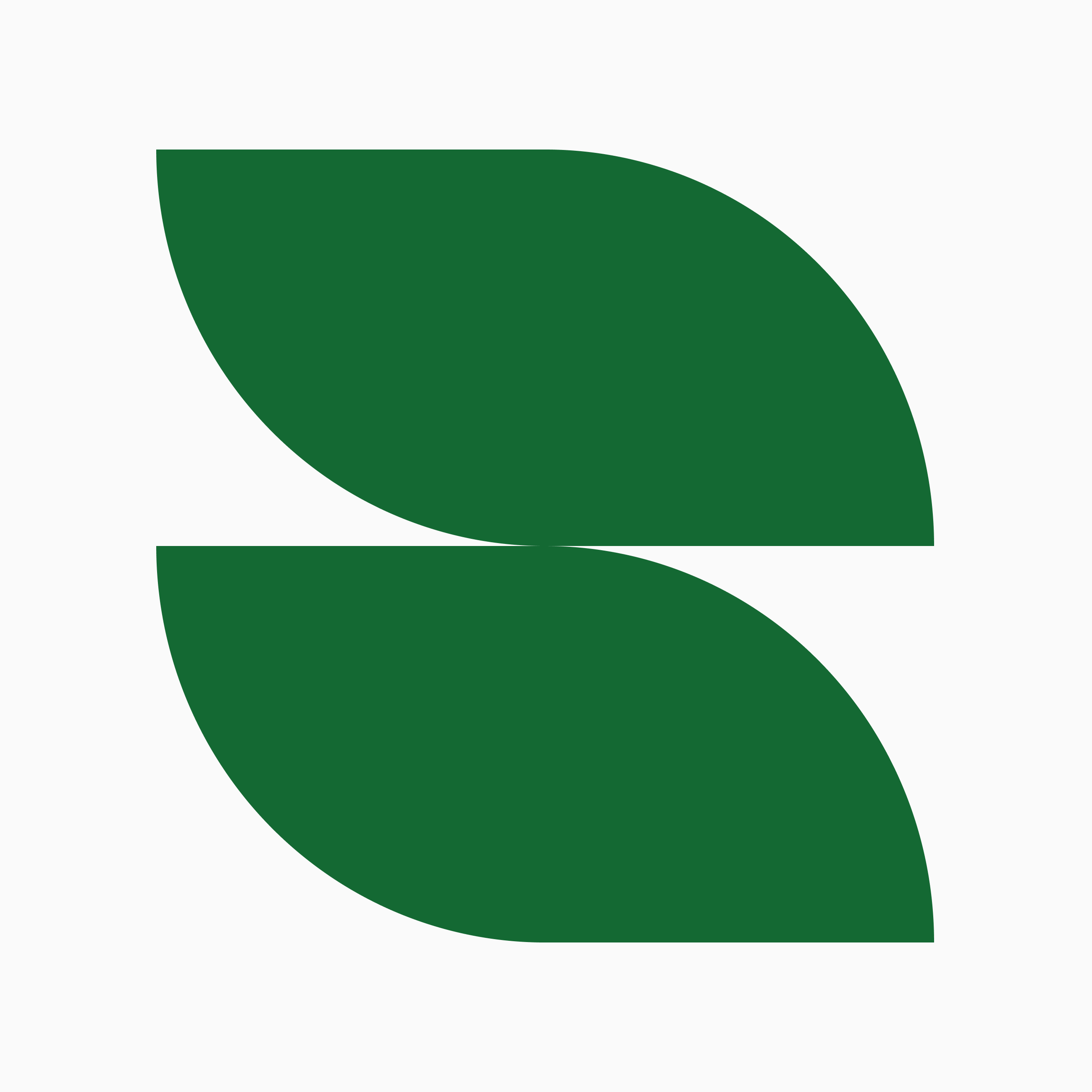
helpy-ui
Find Tools That Cover Your Code
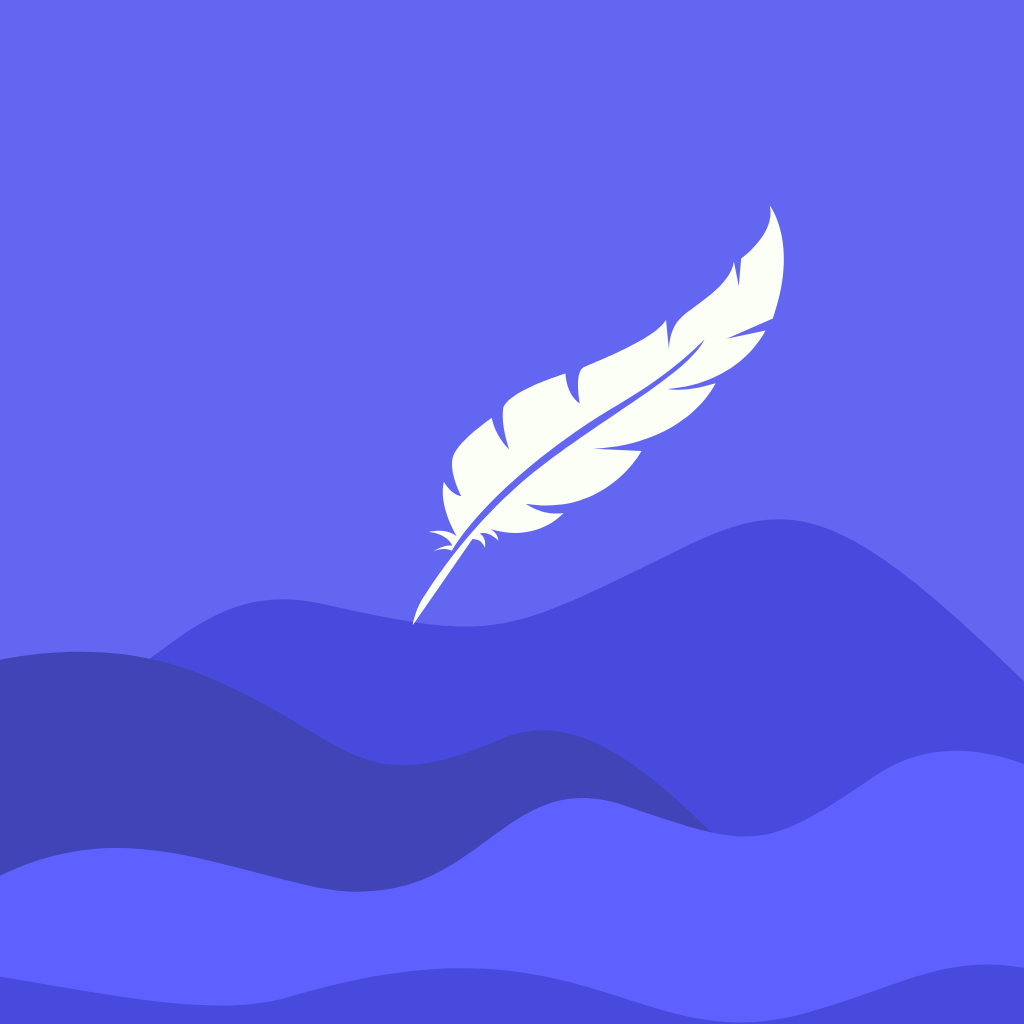
PeakNote: AI Note Taker & Voice Recorder App
AI note taker for voice recording, meeting transcription, and smart summaries

Cloud Infrastructure Platform
Simple, affordable cloud hosting for developers and businesses
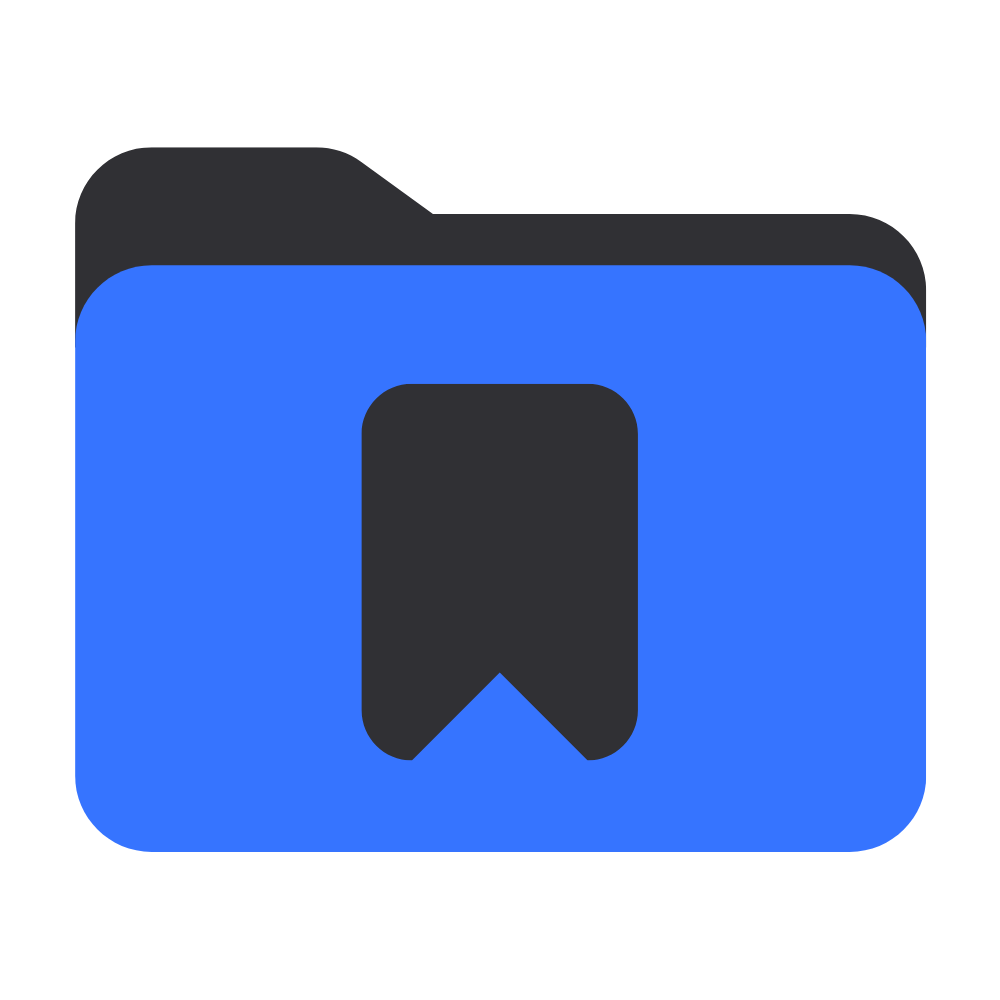
Bookmark Folders for ChatGPT
Search Your ChatGPT History and Manage Your Chats in Folders